A hands On Nodejs
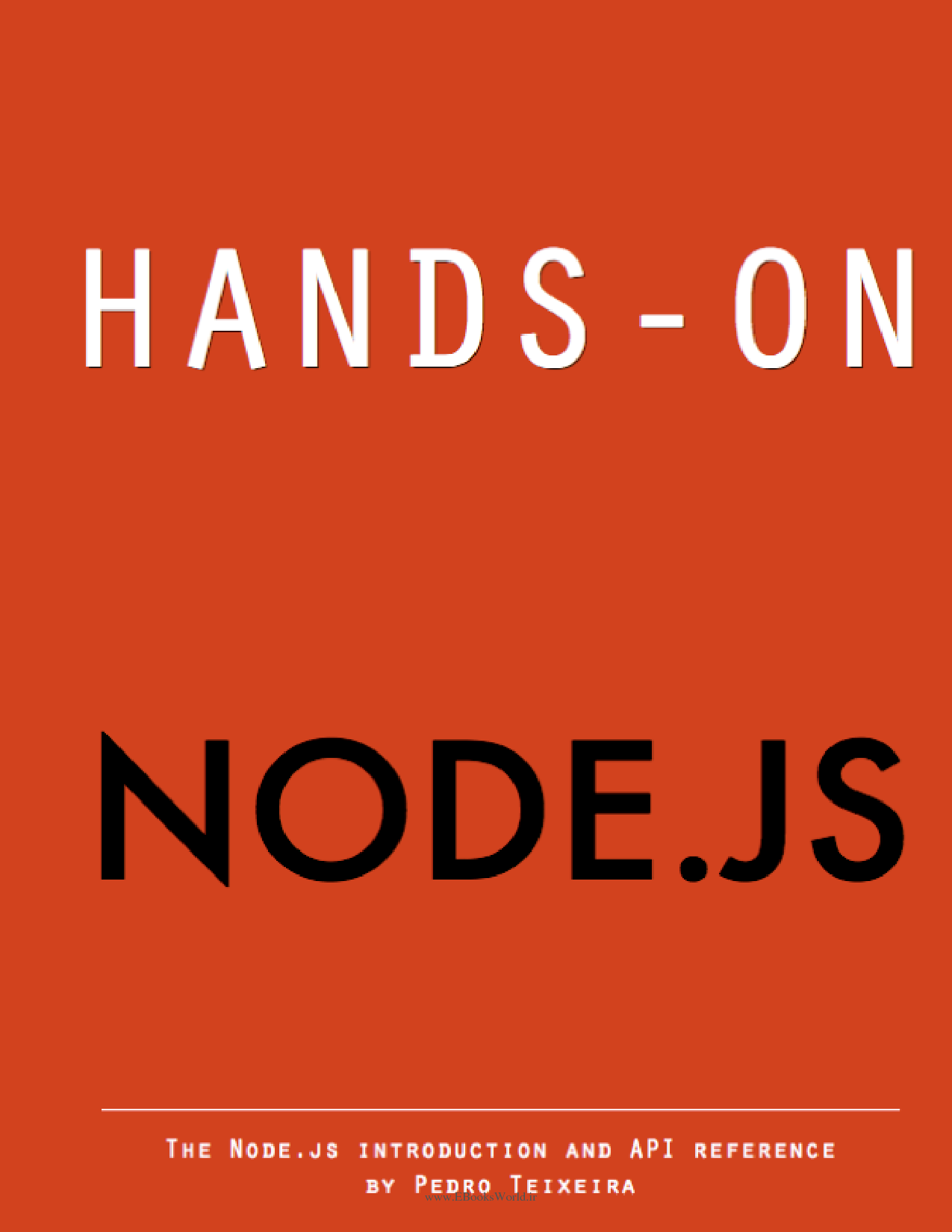
در این کتاب به این خواهیم پرداخت که چرا Node تبدیل به یک موضوع جدید و متفاوت نسبت به دیگر مشابهانش شده است ! به این خواهیم پرداخت که چرا میبایست به فکر استفاده از آن برویم ؟ و چطور با آن آشنا شویم و کار با آن را آغاز کنیم ! در فصول اولیه با پیش نیازات آشنا میشوید و آرام آرام به جلو میروید تا اینکه در انتهای کتاب بایستی توانایی اینکه بتوانید سرویسها و .... را در Node پیاده سازی کنید بدست آورده باشید و همچنین احساس کنید که آشنایی خوبی با API ، Node در طول مطالعهی این کتاب به دانش شما اضافه شده است
نویسندهی کتاب آقای Pedro Teixeira یکی از چهرههای آشنا در زمینهی Nodejs میباشد و چندین اسکرین کست نیز در همین زمینه در وبسایت nodetuts میباشد همچنین وی نویسندهی چندین ماجول در زمینهی نود نیز هست
در ادامه سرفصل کتاب را میتوانید مشاهده کنید :
- Introduction
- Why the sudden, exponential popularity?
- What does this book cover?
- What does this book not cover?
- Prerequisites
- Exercises
- Source code
- Where will this book lead you?
- Why?
- Why the event loop?
- Solution 1: Create more call stacks
- Solution 2: Use event-driven I/O
- Why JavaScript?
- How I Learned to Stop Fearing and Love JavaScript
- Function Declaration Styles
- Functions are first-class objects
- JSHint
- JavaScript versions
- How I Learned to Stop Fearing and Love JavaScript
- References
- Why the event loop?
- Starting up
- Install Node
- Understanding
- Understanding the Node event loop
- An event-queue processing loop
- Callbacks that will generate events
- Don’t block!
- Understanding the Node event loop
- Modules and NPM
- Modules
- How Node resolves a module path
- Core modules
- Modules with complete or relative path
- As a file
- As a directory
- As an installed module
- How Node resolves a module path
- NPM - Node Package Manager
- Global vs. Local
- NPM commands
- npm ls [filter]
- npm install package[@filters]
- npm rm package_name[@version] [package_name[@version] …]
- npm view [@] [[.]…]
- The Package.json Manifest
- Modules
- Utilities
- console
- util
- Buffers
- Slice a buffer
- Copy a buffer
- Buffer Exercises
- Exercise 1
- Exercise 2
- Exercise 3
- Event Emitter
- Creating an Event Emitter
- Event Emitter Exercises
- Exercise 1
- Exercise 2
- Timers
- setTimeout
- clearTimeout
- setInterval
- clearInterval
- setImmediate
- Escaping the event loop
- A note on tail recursion
- Low-level file-system
- fs.stat and fs.fstat
- Open a file
- Read from a file
- Write into a file
- Close Your files
- File-system Exercises
- Exercise 1 - get the size of a file
- Exercise 2 - read a chunk from a file
- Exercise 3 - read two chunks from a file
- Exercise 4 - Overwrite a file
- Exercise 5 - append to a file
- Exercise 6 - change the content of a file
- HTTP
- HTTP Server
- The http.ServerRequest object
- req.url
- req.method
- req.headers
- The http.ServerResponse object
- Write a header
- Change or set a header
- Remove a header
- Write a piece of the response body
- The http.ServerRequest object
- HTTP Client
-
- http.get()
- http.request()
-
- HTTP Exercises
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- HTTP Server
- Streams
- ReadStream
- Wait for data
- Know when it ends
- Pause it
- Resume it
- WriteStream
- Write to it
- Wait for it to drain
- Some stream examples
- Filesystem streams
- Network streams
- The Slow Client Problem and Back-pressure
- What can we do?
- Pipe
- ReadStream
- TCP
- Write a string or a buffer
- end
- …and all the other methods
- Idle sockets
- Keep-alive
- Delay or no delay
- server.close()
- Listening
- TCP client
- Error handling
- TCP Exercises
- Exercise 1
- Exercise 2
- Datagrams (UDP)
- Datagram server
- Datagram client
- Datagram Multicast
- Receiving multicast messages
- Sending multicast messages
- What can be the datagram maximum size?
- UDP Exercises
- Exercise 1
- Child processes
- Executing commands
- Spawning processes
- Killing processes
- Child Processes Exercises
- Exercise 1
- Streaming HTTP chunked responses
-
- A streaming example
- Streaming Exercises
- Exercise 1
-
- TLS / SSL
- Public / private keys
- Private key
- Public key
- TLS Client
- TLS Server
- Verification
- TLS Exercises
- Exercise 1
- Exercise 2
- Exercise 3
- Exercise 4
- Exercise 5
- Public / private keys
- HTTPS
- HTTPS Server
- HTTPS Client
- Making modules
- CommonJS modules
- One file module
- An aggregating module
- A pseudo-class
- A pseudo-class that inherits
- node_modules and npm bundle
- Bundling
- Debugging
- console.log
- Node built-in debugger
- Node Inspector
- Live edit
- Automated Unit Testing
- A test runner
- Assertion testing module
- should.js
-
- Assert truthfulness:
- or untruthfulness:
- = true
- = false
- emptiness
- equality
- equal (strict equality)
- assert numeric range (inclusive) with within
- test numeric value is above given value:
- test numeric value is below given value:
- matching regular expressions
- test length
- substring inclusion
- assert typeof
- property existence
- array containment
- own object keys
- responds to, asserting that a given property is a function:
-
- should.js
- Putting it all together
- Callback flow
- The boomerang effect
- Using caolan/async
- Collections
- Parallel Iterations
- async.forEach
- async.map
- async.forEachLimit
- async.filter
- async.reject
- async.reduce
- async.detect
- async.some
- async.every
- Flow Control
- async.series
- async.parallel
- async.whilst
- async.until
- async.waterfall
- async.queue
- Collections

